Get started with CSP
What is Content Security Policy?
CSP is a security standard that helps prevent various types of attacks by specifying which resources (scripts, styles, images, etc.) are allowed to be loaded and executed on your webpage. Think of it as a whitelist that explicitly states which sources are trusted.Why Use CSP?
CSP helps protect against XSS attacks, clickjacking, code injection, and other security vulnerabilities by controlling which resources can be loaded and executed on your webpage.
Scan Your Website Now
Instantly analyze your website's Content Security Policy. Get actionable insights and improve your security posture in minutes.
Scan Your Website
Enter your website URL to analyze its Content Security Policy configuration.
Get started now by providing your website URL and launch the scan!
Implementation Methods
HTTP Headers
CSP can be implemented through HTTP headers or meta tags. HTTP headers are recommended for better security & compatibility:CSP implementation via HTTP header
Content-Security-Policy: default-src 'self';
Meta Tags
Alternatively, you can use a meta tag in your HTML document:CSP implementation via meta tag
<meta http-equiv="Content-Security-Policy" content="default-src 'self';">
Meta Tag Limitations
While meta tags are convenient, they provide less security than HTTP headers. They can be bypassed if an attacker manages to inject HTML before the meta tag, and they don't support all CSP directives. For production environments, HTTP headers are strongly recommended.
Common Server Configurations
Here's how to implement CSP headers in different server environments:Nginx
Nginx configuration
server {
add_header Content-Security-Policy 'default-src 'self';' always;
}
Nginx Configuration
The 'always' directive ensures the header is sent with every response, including error pages.
Apache
Apache (.htaccess) configuration
Header set Content-Security-Policy 'default-src \'self\';
Express.js
Express.js middleware implementation
app.use((req, res, next) => {
res.setHeader('Content-Security-Policy', 'default-src 'self';');
next();
});
Understanding Nonces
Nonces are crucial for securing inline scripts and styles:Nonce generation in Node.js
const crypto = require('crypto');
const nonce = crypto.randomBytes(16).toString('base64');
Important Implementation Consideration
Nonces must be unique per page load and cryptographically random. Never reuse nonces across requests.
Example of nonce usage
// In your CSP header
Content-Security-Policy: script-src 'nonce-random123';
// In your HTML
<script nonce="random123">
console.log('Hello World');
</script>
Avoid unsafe-inline
Using 'unsafe-inline' defeats much of CSP's security benefits. Always prefer nonces or hashes over unsafe-inline.
Testing Your Implementation
Before enforcing CSP, use Report-Only mode to identify potential issues: Learn how to set up CSP reportingReport-Only mode example
Content-Security-Policy-Report-Only: default-src 'self'; report-uri https://report.centralcsp.com/<myendpoint>;
CSP Violation Monitoring
During development, you can also monitor CSP violations directly in your browser's developer tools: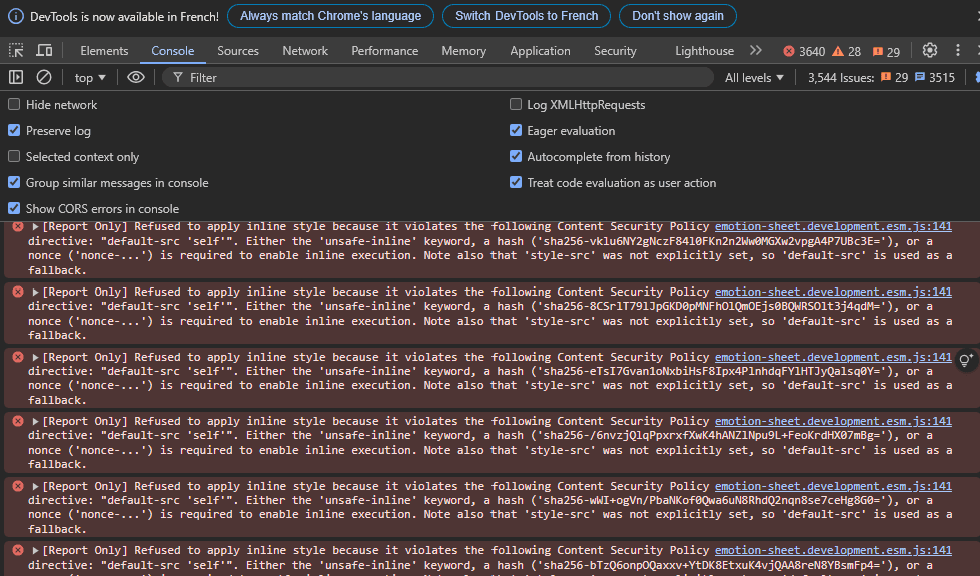
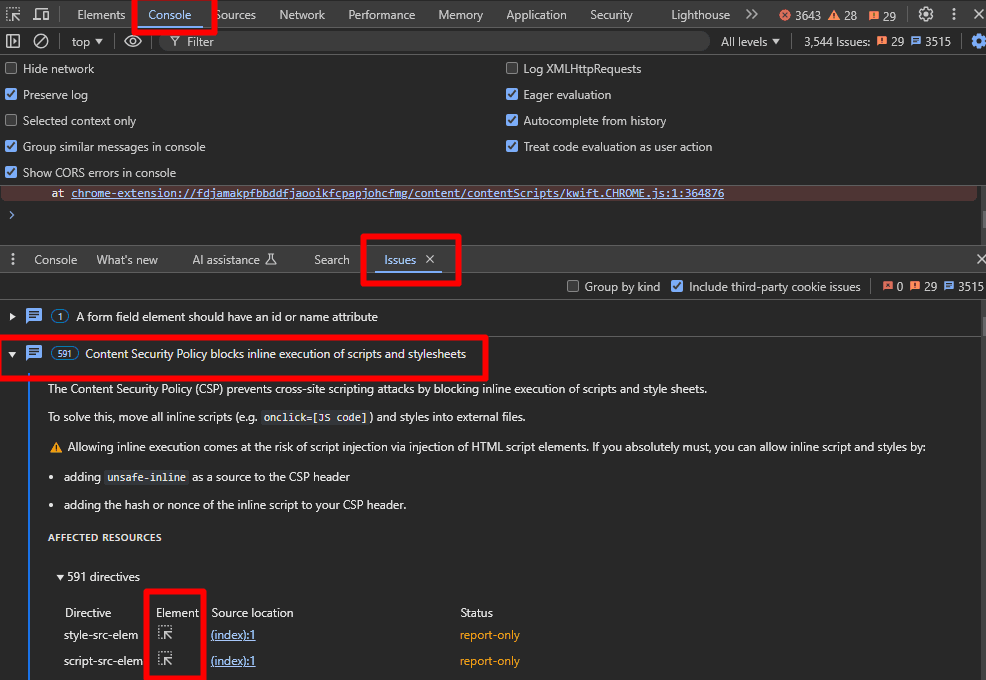
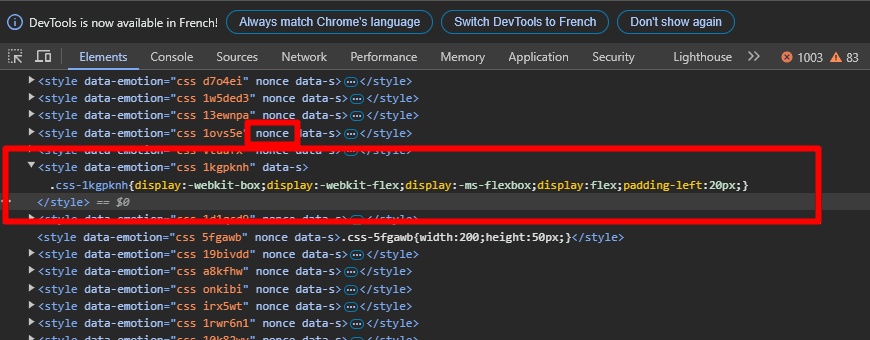
Development Tools
Browser developer tools are great for debugging but don't replace proper CSP reporting in production.
Get Started with CSP reporting
Start securing your website with CSP reporting today
Get Started now with Central CSPBest Practices
Implementation Strategy
Start with Report-Only mode and gradually enforce policies
- Monitor violations before enforcement
- Use nonces instead of unsafe-inline
- Keep policies as restrictive as possible
Security Considerations
Follow security best practices
- Avoid unsafe-inline and unsafe-eval
- Use strict-dynamic where appropriate
- Regularly audit and update policies
Conclusion
Implementing CSP requires careful planning and understanding of your application's architecture. Start with analysis, implement gradually using Report-Only mode, and continuously monitor and adjust your policy as needed.